For making decisions and choosing stuff
Introduction to Logical Arrays
A logical (or BOOLEAN) data type is a data type that can have ONLY two possible values: true
or false
. Logical Arrays are often used to control program flow (e.g. IF ELSE statements) and are extremely useful as binary masks for digital images.
In MATLAB, a logical array has all of the properties of a normal array (it can be indexed and manipulated in exactly the same fashion), but each element can only have one of two values: 0
or 1
, where 0
means false
and 1
means true
.
Fun Fact: The power symbol is simply a one overlaid on top of a zero, where one means “on” and zero means “off”. For more information about the power symbol, see here.
This module is broken down into the following sections:
Learning Objectives
- Define Boolean
- Use the functions true, false, and logical to create logical arrays
- Use Relational Operators to generate logical arrays
- Use Logical Operators to combine logical arrays
- Use logical arrays to index arrays
- use is* functions to detect states and create logical arrays to parse arrays
- Use strcmp on character arrays
Important Terminology
- BOOLEAN – a data type that can have only two possible values
- logical operations – operators that work on the logical class type
- Logical Indexing – using a logical array as an index
- Logic Gates. A function that compares one or more logical inputs and produces a single logical output
Important MATLAB Functions
- true – create Logical
1
- false – create Logical
0
- logical – converts an array to the MATLAB logical class. All non-zero values in the array are converted to
1
’s - strcmp – compare strings
- is* – detect states (e.g. isletter, isspace, isnumeric)
Creating Logical Arrays
Intro | Creating Logical Arrays | Logical Indexing
Explicit Generation or Conversion
TOP || Creating Logical Arrays >> Explicit Generation or Conversion | Relational Operators | Logical Operations
You can explicitly create a logical array using the functions true
or false
>> L = true
L =
1
L
is a 1X1 logical array that takes up 1 byte of memory.
>> whos('L')
Name Size Bytes Class Attributes
L 1x1 1 logical
Adding inputs to the function call, you can create larger matrices of logical arrays using true
or false
…
false(3,4)
…returns a logical matrix with 3 rows and 4 columns of zeros (or false
):
ans =
0 0 0 0
0 0 0 0
0 0 0 0
Relational Operations
TOP || Creating Logical Arrays >> Explicit Generation or Conversion | Relational Operators | Logical Operations
Relational Operations are used to compare values across the elements of different variables. Relational Operators automatically return logical arrays.
Relational Operators
Relational operators compare values and return logical arrays.
Symbol | Relational Function |
---|---|
> | is greater than |
>= | is greater than or equal to |
< | is less than |
<= | is less than or equal to |
== | is equal to |
~= | is not equal to |
The following statement assigns the value 12
to a variable named a
:
a = 12
Whereas this statement compares the contents of the variable a
with the value 12
:
a == 12
If the contents match the value 12
, the operation returns a true
(or a 1
) ; otherwise the operation returns a false
or a 0
. In this case, since we already assigned the value 12
to a
(see previous statement), comparing the contents of a
to the value 12
using the “is equal to” relational operator will return a true
. Since there is no explicit assignment in the second statement, the value true
will be assigned to the variable ans
.
ans =
logical
1
Review ans
now in the workspace. Notice that the logical class requires only 1 byte per element of storage (see the Memory and Numeric Classes for more information on bits and bytes).

Challenge 1
What do you think would happen if you entered the following command in the command window?
a == 13
- Does the value of a change?
- What about the value of
ans
?
How would you assign the output from the relational comparison to a new variable called b
?
see answer
Relational Operation Assignment
We can assign the result of a relational operation to a variable using the following syntax:
>>b = a > 25
b =
0
- In this case, we are assigning the result of the Relational Operation (is
a
is greater than 25?) to a new variableb
. Notice in the workspace thatb
is now a logical data type and that it has the value of0
(orfalse
).
We can similarly compare arrays of data. For example, consider the following relational operation of a vector:
a = 1:10
b = a>5
b =
1×10 logical array
0 0 0 0 0 1 1 1 1 1
b
is a logical array with the same dimensions asa
but with1
’s wherevera
is greater than 5 and0
‘s everywhere else.
Or consider this relational operation of a 2D matrix:
>>c = [1 2 3; 4 5 6]
>>d = c > 3
d =
0 0 0
1 1 1
- The resultant logical array,
d
, has the same dimensions asc
, but has1
’s whereverc
has a value greater than3
. Using this syntax, we can quickly identify which values inc
are greater than3
.
Logical Operations
TOP || Creating Logical Arrays >> Logical Operations | AND | OR |NOT | XOR |
Logical operations are used to combine multiple logical arrays into a single logical array. In brief, logical operations compare the contents of corresponding elements in two logical arrays and returns a logical array of equivalent size in response. For example, consider the following two arrays (array 1 and array 2):
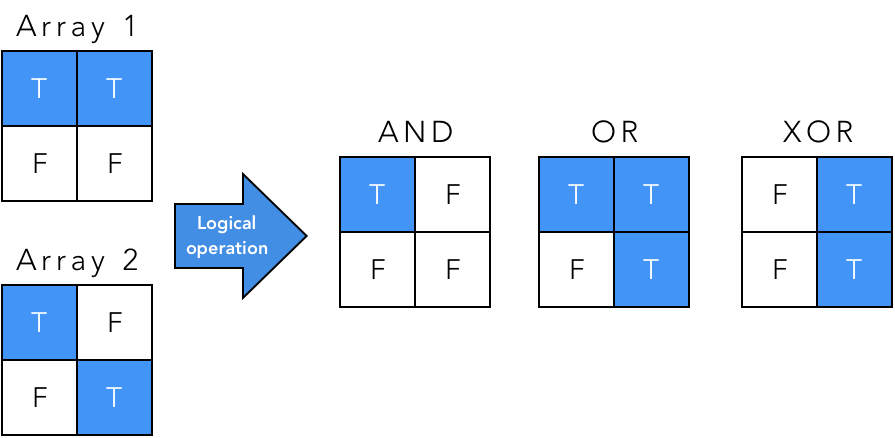
For the logical operation:
- AND: the elements in the output array contain a
true
only where atrue
is found in BOTH of the corresponding elements in the input arrays - OR: the elements in the output array contain a
true
if atrue
can be found in either (or both) of the corresponding elements in the input arrays - XOR: the output contains a
true
only when the corresponding elements contain onetrue
and one false
Please refer to the posted Truth Table for more detail.
Logical Operators
These operators are used in MATLAB syntax to compare logical arrays and return a new logical array
Symbol | Logical operation |
---|---|
& | logical AND |
| | logical OR |
xor | Logical exclusive OR |
~ | logical NOT |
Logical Operations Examples
To understand how logical operations work, consider the following:
First, let’s create a numeric array N
, a 3X3 numeric array incrementing from 1 to 9.
N = reshape(1:9,3,3)
N = 3×3
1 4 7
2 5 8
3 6 9
Then a logical array L
, which has true
(or 1
’s) at the indices where N is greater than 4:
L = N>4
L = 3×3 logical array
0 0 1
0 1 1
0 1 1
…and then a logical array M
, which has true
at the indices where N
is an odd number. Recall the function mod
returns the remainder after division of the dividend (the elements in the variable N
) by the divisor (the number 2
).
>> M = logical(mod(N,2))
M = 3×3 logical array
1 0 1
0 1 0
1 0 1
1: Logical AND
TOP || Creating Logical Arrays >> Logical Operations | AND | OR |NOT | XOR |
The logical AND operation returns true
for coinciding trues and returns false
for anything else. The following AND
relational operation creates a new logical array with true
at indices corresponding to the elements in N
which are both greater than 4 AND are odd:
L&M
ans = 3×3 logical array
0 0 1
0 1 0
0 0 1
- The resultant logical array has a
true
only where there istrue
in bothL
andM
. Thesetrue
’s correspond to the values 5, 7, and 9 inN
: the only values that meet the criteria of being both greater than 4 and odd. The logical array is said to mask those values inN
- You could also break down the logical operation into more simpler steps. The following syntax returns the same logical array as above:
N>4 & logical(mod(N,2))
2: Logical OR
TOP || Creating Logical Arrays >> Logical Operations | AND | OR |NOT | XOR |
The logical OR operation is less discerning than AND: Any true
returns a true
.
The following syntax creates a new logical array with true
at indices corresponding to the elements in N
that are either greater than 4 or odd:
L|M
ans = 3×3 logical array
1 0 1
0 1 1
1 1 1
- The resultant logical array has a
true
wherever there was atrue
found in eitherL
orM
. It has afalse
in the elements corresponding to 2 and 4 inN
. So, 2 and 4 are neither greater than 4 or odd… The rest of the numbers are at least one or the other or both.
3: Logical NOT
This operation is simple to understand: simply invert the logical. For example, the logical NOT of M
is:
~M
ans = 3×3 logical array
0 1 0
1 0 1
0 1 0
- Notice the use of the
tilde
special character. The resultant logical array has the inverse logical ofM
.true
isfalse
andfalse
istrue
. UP is DOWN. CATS are DOGS. etc. - More relevantly, this logical array has
true
at indices corresponding to the elementsN
which are even.
4: Logical XOR
The operation exclusive or is a little more confusing to understand. But the concept is relatively straight forward: You only get a true
from one true
and one false
. Anything else returns a false
. For example:
xor(L,M)
ans = 3×3 logical array
1 0 0
0 0 1
1 1 0
- The resultant logical array masks the odd numbers below 4 and the even numbers above 4:
1, 3, 6, 8
Challenge 2
What syntax returns a logical array that masks even numbers below 4?
Logical Indexing
TOP | Creating Logical Arrays | Logical Indexing
We can use logical arrays as logical indices. This only works when the logical array has the same dimensions as the array being indexed.
For example, consider the following example using the arrays that we generated in the Relational Operations section. Since c
and d
have the same dimensions we can use d
, the logical array, to index c
, the numeric array.
c = [1 2 3; 4 5 6]
d = c > 3
c(d)
ans =
4
5
6
As you can see, doing so returns the values in c
that correspond to the locations in d
where there are 1
’s.

With this syntax, we are essentially saying return all values in c that share the same indices as the 1's in d
. Notice that the result has the same class type (double) as the array is being indexed (c
). MATLAB spits out a vertical vector because the resulting numeric array has fewer elements than the original array and MATLAB doesn’t know how to organize the elements.
Examples using Logical Indexing
1: Logical indexing using Numeric Comparisons
TOP | Logical Indexing >> Example ONE | Example TWO
Consider the Variables L
, N
, and M
that we generated in the previous section. If you index N
with L
as follows…
>> N(L)
ans =
5
6
7
8
9
…you get all the values in N
that are greater than 4
.
If you index N
using the logical array M
…
N(M)
ans =
1
3
5
7
9
…you get all the odd numbers in N
.
2: Logical indexing from combined Logical arrays
TOP | Logical Indexing >> Example ONE | Example TWO
If you index N
using L
AND M
…
N(L&M)
ans =
11
12
13
14
…you get the odd numbers greater than 4.
If you index N
with L
OR M
…
N(L|M)
ans = 7×1
1
3
5
6
7
8
9
…you get all odd numbers and values over four
If you index N
using the XOR of L
and M
N(xor(L,M))
ans =
1
3
6
8
…you get ODD numbers below 4 and even numbers above 4. Or put another way: you get all the values in N
except where L
and M
overlap.
Challenge 3
What syntax indexes N
to return all of the even numbers?
is* Functions
MATLAB has many functions designed to detect states of an array. These functions return logical arrays based on the state of the array. Examples include iscell, ischar, isnumeric and isletter.
Example: isletter
Consider ch
:
>> ch = '1':'Z'
ch =
'123456789:;<=>?@ABCDEFGHIJKLMNOPQRSTUVWXYZ'
The function isletter
returns a logical array containing 1
wherever there is a letter in the input variable. For example:
>> il = isletter(ch)
il =
1×42 logical array
0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1
il
contains 1
’s only where letters can be found in ch
.
If you index ch
with il
…:
>> ch(il)
ans =
'ABCDEFGHIJKLMNOPQRSTUVWXYZ'
…you get the letters from ch
and nothing else.
Example: Pangrams
Pangrams are sentences that contain all of the letters of the alphabet at least once. Let’s use MATLAB to determine whether a sentence is a pangram or not. To do this, we simply need to find all of the unique letters in a sentence and add them up. If they add up to 26, then we have a pangram. There is even a MATLAB function that will return all of the unique characters in a character array: unique
Consider az
:
az = 'The quick brown fox jumps over the lazy dog'
Does this sentence have all of the letters of the alphabet in it (also known as a pangram)? To count the letters, we need to remove the spaces. And to remove the spaces, we first need to find them. For example:
>>sp = isspace(az)
sp =
1×43 logical array
0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 1 0 0 0 0 0 1 0 0 0 0 1 0 0 0 1 0 0 0 0 1 0 0 0
sp
is a logical array that has 1
’s where there are spaces. So, now we can use sp
as a logical index to remove spaces. Remember, the empty brackets?
>>az(sp) = [];
az =
'Thequickbrownfoxjumpsoverthelazydog'
az
now contains no spaces. To count the number of letters, we can use the function numel
, which returns the number of elements in an array:
>>count = numel(az)
count =
35
35? We have too many letters because certain letters are repeated. To find just the unique letters, we can use the function unique
, which returns the unique elements in an array, as follows:
>>az = unique(az)
az =
'Tabcdefghijklmnopqrstuvwxyz'
az
now only contains unique instances of each letter. Let’s count the number of elements using numel
:
>> count = numel(az)
count =
27
27? Ah, yes. Capital T and lowercase t are considered to be different letters since MATLAB is case-sensitive. We need to find all the unique lowercase letters.So first, we convert all letters in az
to lowercase using the function lower
:
>>az =lower(az)
az =
'tabcdefghijklmnopqrstuvwxyz'
Then find the unique instances:
az = unique(az);
az =
'abcdefghijklmnopqrstuvwxyz'
And then count:
>>count = numel(az)
count =
26
count
= 26. There are 26 unique lowercase letters, so, yes, az
is a pangram, which we could encode in another variable:
>> ispangram = count == 26
ispangram =
logical
1
Example: strcmp
The function strcmp is another very useful function. This function determines whether two character arrays are identical.
The following example should illustrate why CANVAS can be so bad at grading open responses such as fill in the Blanks:
Consider these two character arrays:
correct_answer = 'Every good boy does fine'
student_answer = 'Every good boy does fine'
Are these two character arrays identical? Let’s test it:
>>the_same = strcmp(correct_answer, student_answer)
the_same =
0
Why did strcmp return a zero? Because the spaces in the two character arrays did not line up
How can you make CANVAS a better grader? Hint: use the function isspace
We eliminate the spaces by:
- Identify all the spaces using isspace which returns a logical array with 1s where the spaces are
- Using the syntax char_array(logic_array) = [] to eliminate the spaces
- Compare the new character arrays using strcmp
cala = isspace(correct_answer)
sala = isspace(student_answer)
correct_answer(cala) = []
student_answer(sala) = []
strcmp(correct_answer, student_answer)
ans =
1
Now, strcmp returns a 1 indicating that the two strings are the same.
Challenge Answers
Intro | Important Terminology | Creating Logical Arrays | Logical Operations | Logical Indexing
Challenge 1 Answer
- a does not change
- ans changes to zero (false)
b = a==13
Challenge 2 Answer
What syntax returns a logical array that masks even numbers below 4?
~L & ~M
Challenge 3 Answer
N(~M)